What You’ll Need
To build the ESP8266 IFTTT button, you’ll need the following components:
– ESP8266 module (NodeMCU or Wemos D1 mini recommended)
– Momentary push button
– 10K ohm resistor
– Breadboard and jumper wires
– Micro USB cable
– Computer with Arduino IDE installed
Component | Qty | Description |
---|---|---|
ESP8266 module | 1 | Wi-Fi microcontroller |
Push button | 1 | Momentary, normally-open |
10K resistor | 1 | Pull-down resistor |
Breadboard | 1 | For prototyping circuit |
Jumper wires | 5-10 | To make connections |
You can find inexpensive ESP8266 boards like the NodeMCU or Wemos D1 mini for under $10 from many online retailers. These modules have the ESP8266 chip along with a USB serial converter for easy programming. The other components are also easy to find and very affordable.
Setting Up the Hardware
Once you’ve gathered the parts, it’s time to start building the circuit. We’ll connect the push button to one of the ESP8266’s digital input pins. When the button is pressed, it will pull the pin HIGH, which the sketch will detect.
Here are the steps to wire it up:
1. Plug the ESP8266 module into your breadboard. Make sure there is enough space on either side for the other components.
2. Connect one leg of the push button to the ESP’s D1 pin. This will be the pin that gets pulled HIGH when pressed.
3. Connect the other leg of the button to one end of the 10K resistor. This acts as a pull-down to keep the input LOW when the button isn’t pressed.
4. Connect the other end of the resistor to GND on the ESP module.
5. Finally, connect the ESP’s 3V3 pin to the positive rail of the breadboard, and GND to the negative rail. This provides power to the pull-down resistor.
Here is a diagram of the completed circuit:
[Circuit diagram showing ESP8266 connected to pushbutton and pull-down resistor]
Double check that your wiring matches the diagram and there are no loose connections. Once you’ve confirmed everything is hooked up correctly, connect the ESP module to your computer using a micro USB cable.
Programming the ESP8266
With the circuit assembled, it’s time to program the ESP8266 to detect button presses and send web requests to IFTTT. We’ll use the Arduino IDE for this, which has built-in support for many ESP8266 boards.
Installing ESP8266 Support
Before you can program the ESP8266 with Arduino IDE, you need to install support for the board. Here are the steps:
- Open Arduino IDE and go to File > Preferences
- In the “Additional Boards Manager URLs” field, paste the following URL:
http://arduino.esp8266.com/stable/package_esp8266com_index.json
- Click OK to close the Preferences window
- Go to Tools > Board > Boards Manager
- Search for “esp8266” and install the “esp8266 by ESP8266 Community” package
- Once installed, select your ESP8266 board from Tools > Board
If you’re using a NodeMCU board, select “NodeMCU 1.0 (ESP-12E Module)”. For a Wemos D1 mini, choose “LOLIN(WEMOS) D1 R2 & mini”. With ESP8266 support installed, you’re ready to start programming.
The Button Sketch
Here is the complete sketch that detects button presses and sends HTTP requests to IFTTT:
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
const char* ssid = "YOUR_WIFI_SSID";
const char* password = "YOUR_WIFI_PASSWORD";
const char* ifttt_url = "YOUR_IFTTT_URL";
const int button_pin = 5; // D1 on NodeMCU/Wemos D1 mini
void setup() {
pinMode(button_pin, INPUT_PULLUP);
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
void loop() {
if (digitalRead(button_pin) == LOW) {
Serial.println("Button pressed!");
if (WiFi.status() == WL_CONNECTED) {
HTTPClient http;
http.begin(ifttt_url);
int response_code = http.GET();
if (response_code > 0) {
Serial.println("HTTP request sent!");
}
else {
Serial.println("Error sending HTTP request");
}
http.end();
}
delay(1000); // Debounce delay
}
}
Before uploading the sketch, you need to modify a few lines:
1. Change YOUR_WIFI_SSID
to the name of your Wi-Fi network
2. Change YOUR_WIFI_PASSWORD
to your Wi-Fi password
3. Change YOUR_IFTTT_URL
to the URL for your IFTTT Maker applet (we’ll set this up in the next section)
Once you’ve made those changes, upload the sketch to your ESP8266. Open the Serial Monitor and make sure it connects to Wi-Fi successfully. If you see an error, double check your Wi-Fi credentials and make sure the ESP is in range of your router.
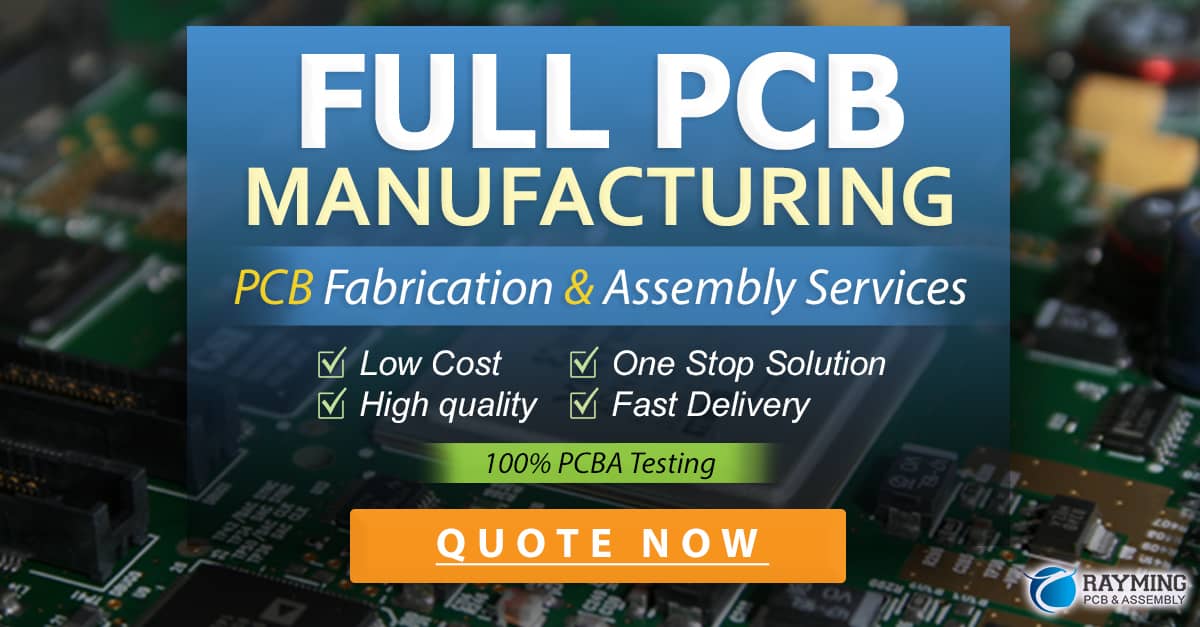
Setting Up IFTTT
To complete the IoT Button, we need to create an applet on IFTTT that will be triggered by the ESP8266. IFTTT is a free web service that lets you create custom automations called “applets”. An applet consists of a trigger (the “if this” part) and an action (the “then that” part).
We’ll use the Maker service for the trigger, which allows you to trigger applets via HTTP web requests. For the action, you can choose from hundreds of services including smart home devices, notification platforms, and more.
Creating a Maker Applet
To create the applet, follow these steps:
1. Sign up for a free account on ifttt.com if you don’t have one already
2. Click your username in the top right and select “Create”
3. Click “Add” next to “If This” and search for “Webhooks”. Select the “Webhooks” service.
4. Choose “Receive a web request” as the trigger
5. Enter an Event Name for your trigger, e.g. “button_pressed”, then click “Create trigger”
6. Now click “Add” next to “Then That” and choose an action service, e.g. “Email”
7. Select the action you want to happen, e.g. “Send me an email”
8. Customize the action fields if needed, then click “Create action”
9. Give your applet a name, then click “Finish”
Your IFTTT button applet is now complete! You can test it by going to the Webhooks documentation page and sending a POST
or GET
request to your trigger URL. The URL will have the following format:
https://maker.ifttt.com/trigger/{event}/with/key/YOUR_KEY
Just replace {event}
with the Event Name you chose in step 5, and YOUR_KEY
with your Maker key which can be found on the Webhooks settings page. If the request is successful, your IFTTT action should be triggered within a few seconds.
Now all that’s left is to update the ifttt_url
variable in your ESP8266 sketch with your IFTTT trigger URL. Upload the sketch again, and press the button to test it out!
Conclusion
Congratulations, you now have a working IoT button that can trigger IFTTT applets! With the power of IFTTT, you can make your button do just about anything. Here are a few ideas to get you started:
- Toggle smart lights or outlets
- Send a notification to your phone
- Log button presses to a Google sheet
- Integrate with Alexa or Google Assistant
- Tweet or post to Facebook
- Start a Roomba vacuum job
- Track work hours in Toggl
The possibilities are endless! Try experimenting with different applets and see what you can come up with.
FAQ
-
Can I use a different type of button?
Yes, you can use any type of momentary push button or switch. Just make sure it’s “normally open” meaning the contacts are disconnected until the button is pressed. -
What if I want to use a different pin on the ESP8266?
You can connect the button to any available GPIO pin. Just change thebutton_pin
variable in the sketch to match the pin number. Keep in mind that some pins have special functions and may not work for this purpose. -
How can I make the button trigger multiple actions?
You can create multiple IFTTT applets with the same trigger event. Each applet can have a different action. Alternatively, you could modify the sketch to send multiple web requests to different IFTTT triggers. -
Can I use the button without IFTTT?
Absolutely! The ESP8266 can send HTTP requests to any web server. You could write your own server-side code to handle the requests and perform any desired actions. -
What’s the range of the ESP8266?
The Wi-Fi range depends on your specific module and environment, but is typically around 50-100 meters indoors. If you need more range, you can connect an external antenna to the ESP8266.
Hopefully this guide has helped you build your own IoT button and opened up a world of possibilities for automation projects. As you can see, the ESP8266 is a versatile little chip that can be used for all sorts of “Internet of Things” applications. Happy making!
0 Comments