Key Features of VHDL
VHDL has several essential features that make it a powerful language for hardware design:
-
Abstraction: VHDL allows designers to describe hardware at various levels of abstraction, from high-level behavioral descriptions to low-level gate-level implementations.
-
Modularity: VHDL supports a modular design approach, enabling the creation of reusable components and facilitating collaboration among design teams.
-
Concurrency: VHDL can describe the parallel nature of hardware, allowing multiple processes to execute simultaneously.
-
Timing: VHDL includes constructs for specifying timing constraints and modeling the timing behavior of digital circuits.
-
Synthesis: VHDL code can be synthesized into a netlist, which is a representation of the circuit’s gate-level implementation.
VHDL Design Units
VHDL code is organized into design units, which are the building blocks of a VHDL model. The main design units in VHDL are:
-
Entity: Defines the interface of a design, specifying input and output ports.
-
Architecture: Describes the internal structure and behavior of a design, implementing the functionality specified in the entity.
-
Package: Contains shared declarations, such as constants, types, and subprograms, that can be used across multiple design units.
-
Configuration: Specifies how design units are connected and configured in a design hierarchy.
Here’s an example of a simple VHDL entity and architecture:
-- Entity declaration
entity example is
port (
a, b : in std_logic;
y : out std_logic
);
end example;
-- Architecture definition
architecture behav of example is
begin
y <= a and b;
end behav;
VHDL Data Types
VHDL provides a rich set of data types to represent and manipulate data in hardware designs. Some commonly used data types include:
-
std_logic: Represents a single-bit signal with nine possible values: ‘U’, ‘X’, ‘0’, ‘1’, ‘Z’, ‘W’, ‘L’, ‘H’, and ‘-‘.
-
std_logic_vector: Represents an array of std_logic elements, used for modeling buses and multi-bit signals.
-
signed and unsigned: Used for representing signed and unsigned integers.
-
integer: Represents integer values.
-
boolean: Represents logical values (true or false).
-
real: Represents floating-point numbers.
Here’s a table summarizing the main VHDL data types:
Data Type | Description |
---|---|
std_logic | Single-bit signal with nine possible values |
std_logic_vector | Array of std_logic elements |
signed | Signed integer |
unsigned | Unsigned integer |
integer | Integer values |
boolean | Logical values (true or false) |
real | Floating-point numbers |
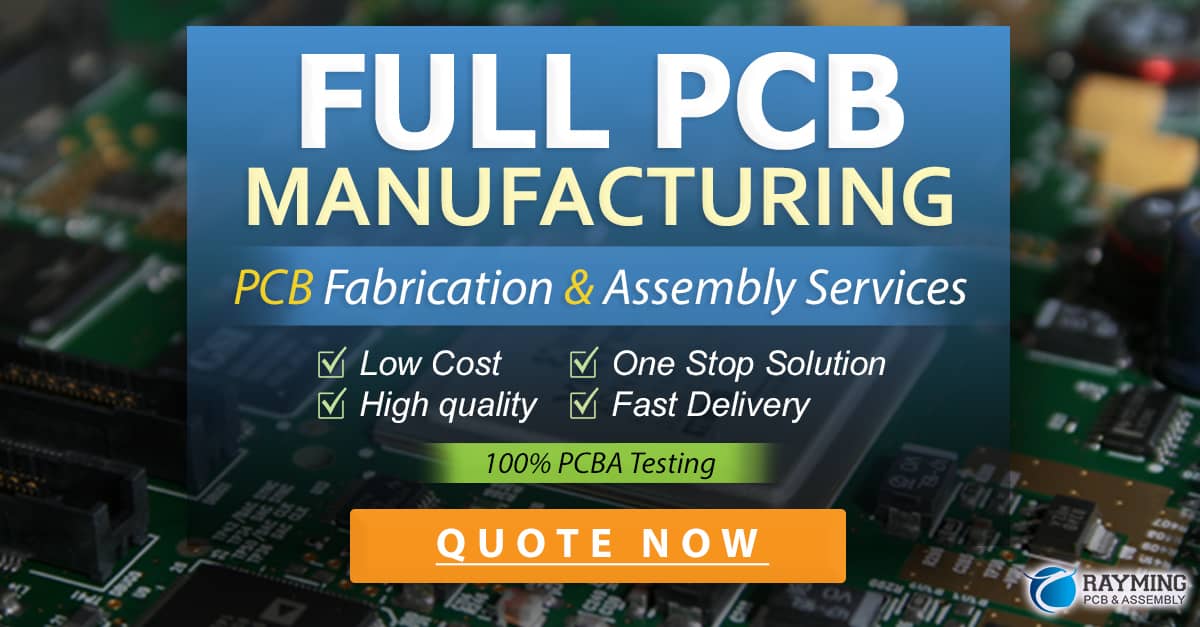
VHDL Operators
VHDL provides a variety of operators for performing operations on data. Some commonly used operators include:
- Logical operators: and, or, nand, nor, xor, xnor, not
- Relational operators: =, /=, <, <=, >, >=
- Arithmetic operators: +, -, *, /, mod, rem
- Concatenation operator: &
- Assignment operator: <=
Here’s an example demonstrating the use of VHDL operators:
architecture behav of example is
signal a, b, c : std_logic;
signal d : std_logic_vector(3 downto 0);
begin
c <= a and b;
d <= "11" & a & b;
end behav;
VHDL Statements
VHDL provides several types of statements for describing the behavior and structure of hardware designs. Some commonly used statements include:
-
Concurrent statements: Executed in parallel and include signal assignments, component instantiations, and process statements.
-
Sequential statements: Executed sequentially within a process and include variable assignments, if statements, case statements, and loop statements.
Here’s an example of a VHDL process with sequential statements:
process(clk)
begin
if rising_edge(clk) then
if reset = '1' then
count <= (others => '0');
else
count <= count + 1;
end if;
end if;
end process;
VHDL Simulation
VHDL designs can be simulated to verify their functionality before synthesis and implementation. A VHDL testbench is used to provide stimulus to the design under test (DUT) and check the outputs for correctness.
Here’s an example of a simple VHDL testbench:
entity tb_example is
end tb_example;
architecture behav of tb_example is
component example is
port (
a, b : in std_logic;
y : out std_logic
);
end component;
signal a, b, y : std_logic;
begin
-- Instantiate the design under test
dut: example port map (a, b, y);
-- Stimulus process
stim_proc: process
begin
-- Test case 1
a <= '0'; b <= '0'; wait for 10 ns;
assert y = '0' report "Test case 1 failed" severity error;
-- Test case 2
a <= '0'; b <= '1'; wait for 10 ns;
assert y = '0' report "Test case 2 failed" severity error;
-- Test case 3
a <= '1'; b <= '0'; wait for 10 ns;
assert y = '0' report "Test case 3 failed" severity error;
-- Test case 4
a <= '1'; b <= '1'; wait for 10 ns;
assert y = '1' report "Test case 4 failed" severity error;
wait;
end process;
end behav;
VHDL Synthesis
After verifying the functionality of a VHDL design through simulation, the next step is to synthesize the code into a gate-level representation. VHDL synthesis tools, such as Xilinx ISE or Intel Quartus Prime, convert the VHDL code into a netlist, which describes the circuit in terms of gates and connections.
During synthesis, the VHDL code is analyzed, optimized, and mapped to the target technology library. The synthesis tool performs tasks such as:
- Parsing and analyzing the VHDL code for syntax and semantic errors.
- Optimizing the design by removing redundant logic and simplifying expressions.
- Mapping the design to the available resources in the target technology library.
- Generating a netlist representing the gate-level implementation of the design.
The synthesized netlist can then be used for further steps in the hardware design flow, such as place and route, timing analysis, and bitstream generation.
VHDL Design Flow
The typical VHDL design flow involves the following steps:
-
Design Entry: Create the VHDL code for the design using a text editor or an integrated development environment (IDE).
-
Simulation: Verify the functionality of the design using a VHDL simulator. Create a testbench to provide stimulus and check the outputs.
-
Synthesis: Synthesize the VHDL code into a gate-level netlist using a synthesis tool.
-
Place and Route: Perform placement and routing of the synthesized netlist onto the target device, such as an FPGA or ASIC.
-
Timing Analysis: Verify that the design meets the required timing constraints, such as setup and hold times.
-
Bitstream Generation: Generate the final bitstream file that can be used to program the target device.
-
Hardware Verification: Test the design on the actual hardware to ensure it functions as intended.
Here’s a table summarizing the main steps in the VHDL design flow:
Step | Description |
---|---|
Design Entry | Create the VHDL code for the design |
Simulation | Verify the functionality using a VHDL simulator |
Synthesis | Synthesize the VHDL code into a gate-level netlist |
Place and Route | Perform placement and routing onto the target device |
Timing Analysis | Verify that the design meets timing constraints |
Bitstream Generation | Generate the final bitstream file for programming |
Hardware Verification | Test the design on the actual hardware |
VHDL vs. Verilog
VHDL and Verilog are the two most widely used hardware description languages in the industry. While both languages serve the same purpose of describing and designing digital circuits, they have some differences in syntax and features.
Here’s a comparison table between VHDL and Verilog:
Feature | VHDL | Verilog |
---|---|---|
Typing | Strongly typed | Weakly typed |
Syntax | More verbose, ADA-like syntax | More concise, C-like syntax |
Popularity | Widely used in Europe and military/aerospace | Widely used in Asia and consumer electronics |
Modeling Style | Dataflow, behavioral, structural | Dataflow, behavioral, structural |
Multi-Valued Logic | Supported (std_logic) | Supported (wire, reg) |
Object-Oriented | Supported (VHDL-2008) | Not natively supported |
Testbench Functionality | Assertion statements, file I/O | Display statements, file I/O |
Despite their differences, both VHDL and Verilog are capable of describing and designing complex digital systems effectively. The choice between the two often depends on the specific project requirements, company or industry preferences, and the designer’s familiarity with the language.
Frequently Asked Questions (FAQ)
-
What is the purpose of VHDL?
VHDL is used to model, design, and simulate digital circuits and systems. It allows engineers to describe the structure and behavior of electronic systems before physically building them. -
Is VHDL case-sensitive?
Yes, VHDL is case-insensitive. This means that keywords, identifiers, and names can be written in uppercase, lowercase, or mixed case, and the VHDL compiler will treat them the same way. -
What is the difference between a signal and a variable in VHDL?
In VHDL, a signal represents a wire or a connection between components and is used for modeling communication between concurrent elements. A variable, on the other hand, is used for temporary storage and computation within a process and is not visible outside the process. -
Can VHDL be used for analog circuit design?
VHDL is primarily used for digital circuit design. However, VHDL-AMS (Analog and Mixed-Signal) is an extension of VHDL that supports the modeling and simulation of analog and mixed-signal circuits. -
What is the role of a VHDL testbench?
A VHDL testbench is a separate entity used to provide stimulus to the design under test (DUT) and verify its functionality through simulation. It instantiates the DUT, generates input signals, and checks the output signals against expected values to ensure the design behaves correctly.
In conclusion, VHDL is a powerful hardware description language used for modeling, designing, and simulating digital circuits and systems. Its key features, such as abstraction, modularity, and concurrency, make it an essential tool in the hardware design process. By understanding the fundamentals of VHDL, including design units, data types, operators, and statements, engineers can effectively create and verify complex digital designs before physically implementing them.
0 Comments