Introduction to Assembly Language
Assembly language is a low-level programming language that has a strong correlation to the architecture of the computer on which it runs. Each assembly language is specific to a particular computer architecture and operating system.
Assembly code consists of mnemonic opcodes and operands. The opcodes are human-readable representations of the machine code instructions executed by the CPU. Operands refer to data or addresses used by the instructions.
Unlike high-level languages like C and Java, assembly language does not utilize variables or complex expressions. It works directly with CPU registers, memory addresses, and other low-level constructs. This gives the programmer precise control over the system’s hardware.
Advantages of Assembly Language
- Speed: Assembly code runs much faster than high-level code since it has a one to one mapping with the native machine code. There is no overhead from abstraction layers.
- Size: Assembly programs are compact because instruction sizes directly map to processor opcodes. This is crucial for embedded and system programming.
- Control: Assembly provides full access to all processor features and executes precisely as intended without unpredictable optimizations.
- Security: The low-level nature of assembly makes it ideal for implementing secure systems, cryptographic algorithms and anti-debugging.
Disadvantages of Assembly Language
- Complexity: Assembly code is more difficult to write and maintain due to its lack of high-level features. Programs require greater programmer effort and skill.
- Portability: Assembly programs only run on the architecture they target. Supporting multiple platforms requires significant rewriting.
- Tooling: Modern high-level languages have abundant libraries, frameworks, and development tools. Assembly has far fewer resources.
- Undefined behavior: Errors result in unpredictable crashes rather than exceptions. Debugging assembly code is challenging.
Key Concepts in Assembly Language
To write assembly code, we need to understand some key low-level concepts:
Registers
Registers are small storage units directly inside the CPU that hold operands involved in computations. Common registers include the accumulator (AX), base (BX), counters (CX, DX), stack pointer (SP), and instruction pointer (IP). The availability and size of registers depend on the target architecture.
Memory Segments
Assembly organizes memory into segments like the stack, heap, data, code and more. We reference memory locations using segment registers like DS for data. Special address registers like BP and SP point to important positions in the stack segment.
Flags
Status flags record the results of operations and conditions like carries, overflows, zeros, sign bits and parity errors. Conditional branching relies on inspecting flags set by instructions like CMP to alter program flow.
Addressing Modes
These specify how operands are accessed. Immediate mode directly encodes values in instructions. Register mode uses registers as operands. Memory addressing modes access operands at memory addresses using techniques like direct, indirect, indexed and relative addressing.
Subroutines
Reusable subroutines implement procedures that are called from the main program. The CALL and RET instructions are used to enter and exit subroutines which receive parameters in registers. This avoids code duplication.
Interrupts
Interrupts alter sequential program flow when events like hardware signals, faults and traps occur. Interrupt service routines (ISRs) invoked handle the events before resuming normal execution. This facilitates interaction with peripherals and multitasking.
Learning x86 Assembly Language
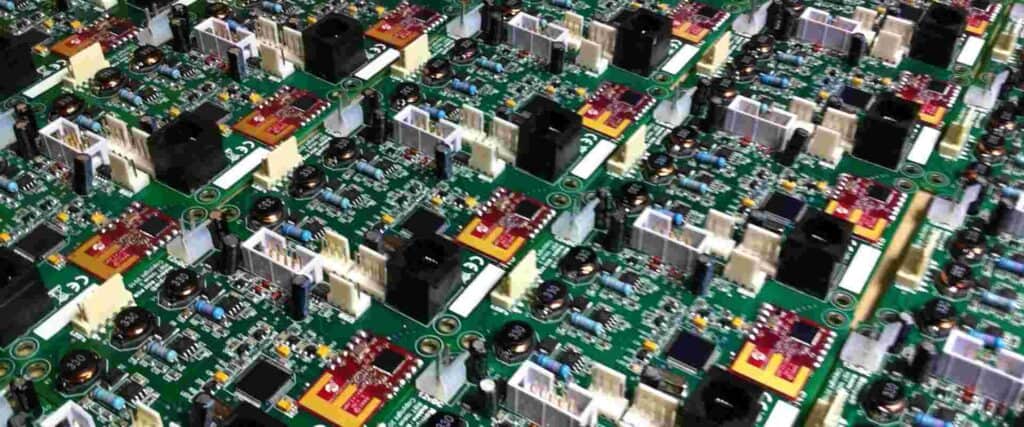
The x86 architecture assembly language is the most commonly used low-level language for Windows, Linux and other mainstream platforms. Let’s go through some key steps to learn it:
1. Choose an Assembler
Assemblers convert assembly code into machine code. Some popular open source x86 assemblers are:
- NASM (Netwide Assembler) – Well documented, supports common formats
- YASM (Yet Another Simplified Assembler) – Very fast, uses NASM syntax
- FASM (Flat Assembler) – More flexible, supports macros and includes
2. Learn the Syntax
The basic assembly structure contains:
- Instructions like MOV, ADD, CALL
- Operands like registers, addresses, immediates
- Directives forcompile-time commands like section, bits, ends
- Comments start with a semicolon (;)
Labels ending with a colon can be referenced by jump instructions.
assembly
Copy code
; Sample syntax mov eax, 10 ; Instruction to load immediate value ret ; Return from subroutine global start ; Assembler directive ; Data declaration data: num dd 500 start: ; Code section mov dx, [num] ; Load memory address add dx, 2 ; Integer addition
3. Understand Data Types
The main data types are:
- BYTE – unsigned 8 bit integer
- WORD – unsigned 16 bit integer
- DWORD – unsigned 32 bit integer
- QWORD – unsigned 64 bit integer
We also have types for signed integers, floats, doubles and other special formats.
4. Use System Calls
To perform tasks like I/O, memory allocation and string manipulation, we need interfaces to the operating system like:
- DOS interrupts on older systems
- SYSCALL instruction on Linux
- WINAPI library on Windows
This involves pushing parameters into registers and triggering interrupts, traps or calls.
5. Learn Debugging
Debuggers let us step through code, inspect registers and memory, set breakpoints, and more. Some debugging options are:
- GDB – The GNU debugger
- Visual Studio debugger
- Debugging information in assembly with directives like .debug_frame
This helps catch bugs during the development process.
6. Write Programs
With the basics covered, we can start writing full assembly programs that use:
- Loops, branches and subroutines
- Macros, includes and other preprocessor features
- Interfaces to system libraries and drivers
- All available instruction sets and addressing modes
Practice is key to mastering assembly language.
Uses of Assembly Language
Now that we understand the key concepts of assembly language, let’s look at some of its major uses:
1. Operating System Kernels
Operating system kernels like Windows and Linux require low-level hardware access. Much of their core is implemented in assembly language for precise control and performance.
2. Device Drivers
Drivers interact closely with hardware so assembly is commonly used, especially in time-critical interrupt handlers. Many compilers can inline assembly code in C driver source.
3. Embedded Systems
Resource-constrained microcontrollers in embedded devices like home appliances, IoT sensors, and vehicles extensively use assembly language. The availability of memory and processing power is limited.
4. Cryptography
Performance-intensive cryptography algorithms rely on assembly language. Functions like encryption and hashing need to process rapidly without latency. Tight hardware integration via assembly maximizes speed.
5. Malware Analysis
Disassembling malware samples is useful for analysis and detection. Observation of suspicious low-level behavior provides insight into malware operation. Automated static and dynamic analysis of assembly code helps security researchers.
6. Reverse Engineering
Reconstructing proprietary software internals through disassembly is common during reverse engineering. Assembly gives the complete picture of program structure unaffected by compiling and linking.
7. Code Optimization
Assembly inserts help optimize performance-critical routines in high-level programs. Vectorization uses SIMD instructions. Loop unrolling, inlining, and branch prediction improves hot code paths.
8. Bootloaders
The boot sequence relies on assembly language. The BIOS, bootloader, and initial kernel phases run before the OS loads. These components initialize hardware and kickstart high-level software.
9. Software Protection
Assembly implements techniques like code obfuscation, anti-debugging, and packing to protect against tampering and piracy. This is applied heavily in DRM schemes.
Key Differences Between Assembly and C
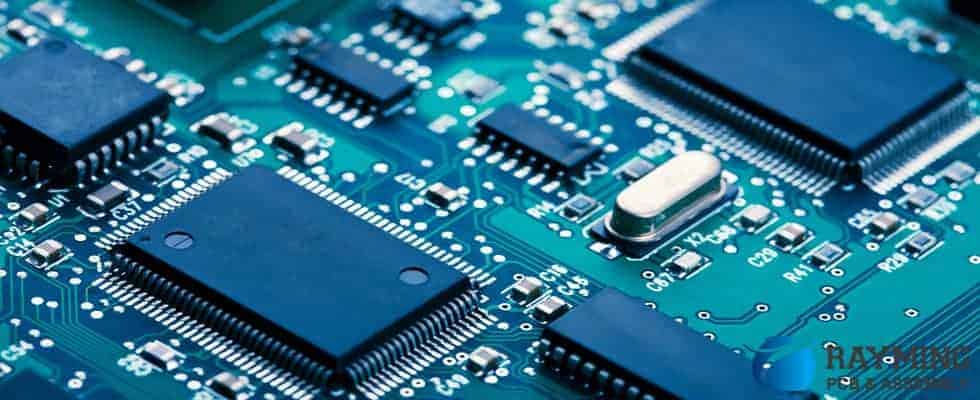
C is a high-level language supporting many features not found in assembly:
1. Portability
C code can compile on various architectures by reusing most of the code. Assembly must be rewritten for each target.
2. Memory Management
C abstracts memory with automatic variables and dynamic allocation. Assembly directly manipulates registers, the stack, and memory addresses.
3. Data Types
C has user-defined types like structs along with integer, float, enum types. Assembly primarily uses machine-specific types matching hardware registers and memory.
4. Syntax and Readability
Assembly uses symbolic opcodes with lots of registers and addresses. C has a concise, rich syntax resembling English.
5. Development Tools
C has many mature open source and commercial tools for editing, building, testing and debugging. Assembly tools are fewer and more fragmented.
6. Safety and Security
C is vulnerable to buffer overflows, dangling pointers, and other defects requiring manual discipline. Assembly’s simplicity avoids classes of defects but is not immune to programmer error.
7. Libraries and Frameworks
C links into standard system libraries and third party components. Assembly relies on less standardized OS interfaces.
8. Productivity and Maintenance
C has higher level abstractions allowing faster development and easier maintenance. Assembly requires greater programmer effort and skill for complex projects.
Key Aspects of Systems Programming
Systems programming focuses on implementing core OS components like kernels, drivers, servers and embedded firmware. Let’s go through some hallmarks of systems code:
1. Hardware Interaction
Direct access to memory, I/O devices, interrupts and processor features is needed. This requires in-depth hardware knowledge and APIs.
2. Performance Sensitivity
Latency and throughput are crucial. Solutions optimize for the fastest possible execution using micro-benchmarks.
3. Reliability and Stability
Rigorous interfaces, error handling, protections, and testing ensures the system withstands all conditions and dodges flaws that lead to crashes or hangs.
4. Concurrency and Parallelism
Systems juggle many simultaneous threads, processes, interrupts, asynchronous logic, and inter-process communication. Locking, synchronization and message passing are vital.
5. Resource Management
Efficient memory allocation, disk access, networking and other resource usage minimizes waste. Careful control prevents resource exhaustion.
6. Security
Defenses like access controls, sandboxing, encryption and validation protect from compromise. Vulnerabilities are mitigated or confined.
Key Challenges of Systems Programming
Systems programming is demanding due to these complexities:
1. Intricate Hardware Modeling
Developers must grok the complete hardware environment: buses, memory topology, boot process, instruction sets, and odd corner cases. Missing details cause problems.
2. Pointer and Memory Errors
Usage of raw pointers for direct memory access risks crashes from illegal accesses, leaks, and trampling data. Protecting memory is hard.
3. Timing Dependencies
Race conditions from improper serialization plague multi-threaded code. Meticulous locking and testing required to avoid intermittent glitches.
4. I/O Error Handling
I/O device failures are common. Robust error handling avoids crashes and hangs when peripherals or connections fail. Users see only graceful degradation.
5. Performance Tuning
Systems code sees heavy usage so inefficient routines deteriorate performance. Optimization and profiling required to speed up hot paths and eliminate waste.
6. Vulnerability Management
Flaws like buffer overflows and race conditions are unavoidable. Designing damage containment and applying patches is essential.
Conclusion
In summary, assembly language remains deeply relevant for high performance scenarios like operating systems and embedded devices despite the wide adoption of high-level languages. It provides an unparalleled level of hardware control and efficiency at the cost of greater complexity. Mastering assembly language requires diligence but unlocks capabilities beyond high-level code. The future will continue to demand expertise in this foundational language.
Frequently Asked Questions
Q1: Is learning assembly language still worthwhile today?
A1: Yes, assembly language retains many vital uses today. It underpins hardware interfaces, speeds up performance-critical routines, enables precise control requirements, and supports reverse engineering. Assembly mastery is still valued in systems programming.
Q2: Can C completely replace assembly language?
A2: C cannot fully replace assembly but reduces the need for it significantly. C requires assembly glue for OS, driver and boot code. Optimized libraries use assembly. Some areas like embedded systems and malware analysis still rely heavily on assembly.
Q3: How difficult is assembly language compared to modern languages?
A3: Assembly language is more difficult due to its low-level nature. It lacks many high-level conveniences, requires manual memory management, and has limited tools. Patience is needed to work through complexity and avoid subtle bugs.
Q4: Is assembly language used for artificial intelligence or machine learning?
A4: No, high-level languages like Python and C++ dominate AI/ML due to extensive libraries. Assembly may optimize inner loops for execution speed but is unsuited to productivity-driven development.
Q5: What are the easiest ways to get started with assembly language?
A5: Start by choosing an assembler like NASM, then learn the syntax, data types, and debugger for a beginner-friendly architecture like x86 or ARM. Write small example programs before tackling larger projects. Use available references.
0 Comments