What is a Mouse Rotary Encoder?
A mouse rotary encoder is an electromechanical device that consists of a rotating shaft and a fixed sensing element. As the shaft rotates, the sensing element generates a series of pulses that can be interpreted by a microcontroller or other electronic devices to determine the position, direction, and speed of rotation.
Types of Mouse Rotary Encoders
There are two main types of mouse rotary encoders:
-
Mechanical Rotary Encoders: These encoders use physical contacts to generate electrical signals. They typically consist of a metal disc with a pattern of conductive and non-conductive segments, and a set of brushes or contacts that press against the disc as it rotates.
-
Optical Rotary Encoders: These encoders use light sensors to detect the motion of a patterned disc or strip. They are more reliable and have a longer lifespan than mechanical encoders, as they do not rely on physical contacts that can wear out over time.
How Does a Mouse Rotary Encoder Work?
A mouse rotary encoder works by generating a series of pulses as the shaft rotates. These pulses are typically in the form of two square wave signals, called Channel A and Channel B, which are offset by 90 degrees (quadrature encoding).
Quadrature Encoding
Quadrature encoding allows the microcontroller to determine both the direction and magnitude of rotation. By comparing the relative phase of the two channels, the microcontroller can determine whether the shaft is rotating clockwise or counterclockwise.
Channel A | Channel B | Direction |
---|---|---|
0 | 0 | No movement |
0 | 1 | Clockwise |
1 | 0 | Counterclockwise |
1 | 1 | No movement |
Resolution
The resolution of a mouse rotary encoder is determined by the number of pulses generated per revolution of the shaft. Higher resolution encoders can detect smaller increments of motion, resulting in more precise position tracking.
Connecting a Mouse Rotary Encoder to an Arduino
To connect a mouse rotary encoder to an Arduino, you will need the following components:
- Arduino board (e.g., Arduino Uno)
- Mouse rotary encoder
- Breadboard
- Jumper wires
Step 1: Identify the Encoder Pins
A typical mouse rotary encoder has three pins:
- Ground (GND)
- Channel A
- Channel B
Some encoders may have additional pins, such as a power supply pin (VCC) or a push-button switch pin.
Step 2: Connect the Encoder to the Arduino
- Connect the GND pin of the encoder to the GND pin on the Arduino.
- Connect Channel A of the encoder to a digital input pin on the Arduino (e.g., pin 2).
- Connect Channel B of the encoder to another digital input pin on the Arduino (e.g., pin 3).
If your encoder has a VCC pin, connect it to the 5V pin on the Arduino. If it has a push-button switch pin, you can connect it to another digital input pin on the Arduino if desired.
Step 3: Write the Arduino Code
To read the pulses from the mouse rotary encoder, you will need to write a sketch in the Arduino IDE. Here’s a simple example that demonstrates how to read the encoder and print the rotation direction and count to the serial monitor:
#define ENCODER_A 2
#define ENCODER_B 3
volatile int encoderCount = 0;
volatile bool encoderDir = true;
void setup() {
pinMode(ENCODER_A, INPUT_PULLUP);
pinMode(ENCODER_B, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(ENCODER_A), encoderISR, CHANGE);
Serial.begin(9600);
}
void loop() {
Serial.print("Count: ");
Serial.print(encoderCount);
Serial.print(" Direction: ");
Serial.println(encoderDir ? "Clockwise" : "Counterclockwise");
delay(100);
}
void encoderISR() {
if (digitalRead(ENCODER_A) == digitalRead(ENCODER_B)) {
encoderCount++;
encoderDir = true;
} else {
encoderCount--;
encoderDir = false;
}
}
In this example, we define two constants (ENCODER_A
and ENCODER_B
) to represent the digital input pins connected to the encoder channels. We also declare two volatile variables (encoderCount
and encoderDir
) to store the current count and direction of rotation.
In the setup()
function, we configure the encoder pins as inputs with internal pull-up resistors and attach an interrupt to Channel A. The interrupt service routine (ISR) encoderISR()
is called whenever there is a change in the state of Channel A. Inside the ISR, we compare the states of Channel A and Channel B to determine the direction of rotation and increment or decrement the encoderCount
accordingly.
In the loop()
function, we simply print the current count and direction to the serial monitor every 100 milliseconds.
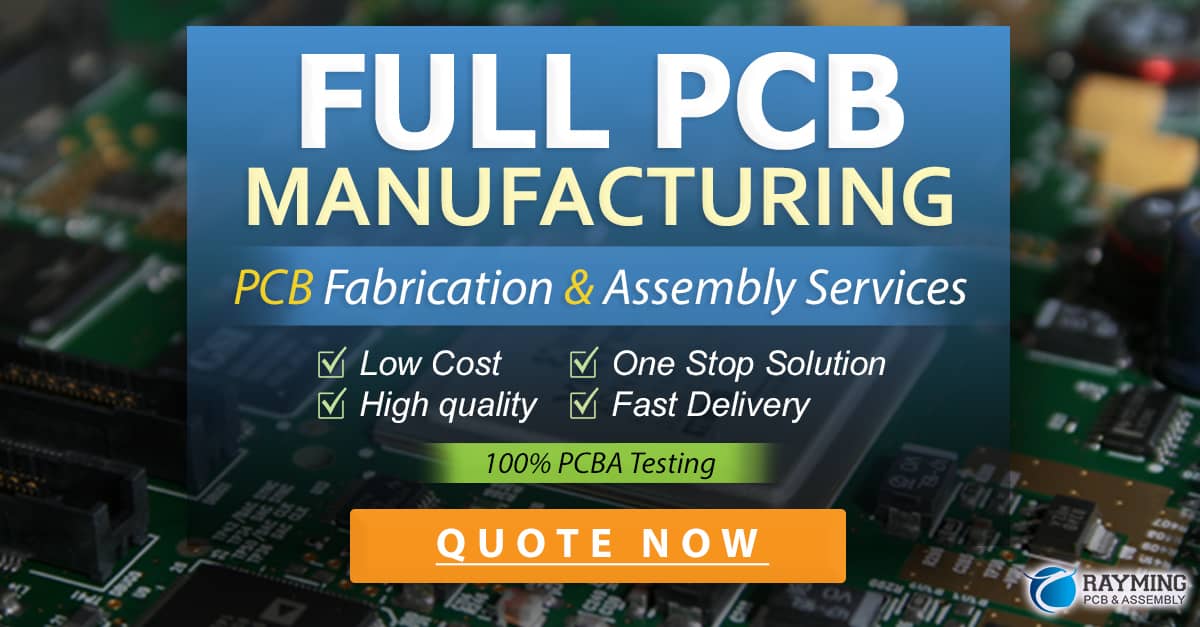
Applications of Mouse Rotary Encoders
Mouse rotary encoders are used in a wide range of applications, including:
- Computer mice and trackballs
- Industrial control systems
- Robotics and automation
- Medical devices
- Audio and video equipment
By providing precise position and motion tracking, mouse rotary encoders enable these devices to operate with high accuracy and reliability.
Frequently Asked Questions (FAQ)
-
What is the difference between a mouse rotary encoder and a potentiometer?
A mouse rotary encoder generates digital pulses to indicate rotation, while a potentiometer is an analog device that provides a variable resistance based on its angular position. -
Can I use a mouse rotary encoder for absolute position sensing?
No, mouse rotary encoders are incremental encoders that only provide relative position information. For absolute position sensing, you would need an absolute encoder or a different type of sensor. -
How do I increase the resolution of my mouse rotary encoder?
The resolution of a mouse rotary encoder is fixed and determined by its physical construction. To increase the resolution, you would need to use a higher-resolution encoder or implement additional signal processing techniques in software. -
Can I connect multiple mouse rotary encoders to a single Arduino?
Yes, you can connect multiple encoders to an Arduino by using different digital input pins for each encoder and creating separate ISRs and variables to track their states. -
Are there any limitations to using mouse rotary encoders?
Mouse rotary encoders have a limited rotational range (typically less than 360 degrees) and may be subject to mechanical wear over time. They also require additional components (e.g., debounce circuits) to filter out noise and ensure reliable operation.
Conclusion
Mouse rotary encoders are essential components in many electronic devices, providing accurate and reliable position and motion tracking. By understanding the working principle of these encoders and how to connect them to a microcontroller like the Arduino, you can harness their capabilities for your own projects and applications.
With the information provided in this article, you should now have a solid foundation in mouse rotary encoders and be able to integrate them into your designs with confidence. As you continue to explore the world of sensors and microcontrollers, keep an eye out for new and innovative ways to use these versatile components.
0 Comments