What is the DS1307?
The DS1307 is an 8-pin, serial I2C real-time clock IC manufactured by Maxim Integrated (formerly Dallas Semiconductor). It is designed to provide accurate timekeeping while consuming minimal power, making it suitable for battery-powered applications. The IC includes a built-in crystal oscillator and can be powered by an external battery, ensuring that timekeeping continues even when the main power supply is disconnected.
Key Features of the DS1307
- Accurate timekeeping: The DS1307 maintains seconds, minutes, hours, day, date, month, and year information, with leap-year compensation valid up to 2100.
- Low power consumption: The IC operates at less than 500nA in battery backup mode, making it ideal for low-power applications.
- I2C interface: The DS1307 communicates via a simple two-wire I2C interface, allowing easy integration with microcontrollers and other devices.
- Programmable square-wave output: The IC provides a programmable square-wave output signal for various applications, such as generating interrupts or driving other devices.
- 56-byte non-volatile RAM: The DS1307 includes 56 bytes of non-volatile RAM for storing user data, which remains available even when the main power supply is disconnected.
DS1307 Pinout
The DS1307 comes in an 8-pin DIP (Dual Inline Package) or an 8-pin SOIC (Small Outline Integrated Circuit) package. The pinout for both packages is identical:
Pin | Name | Description |
---|---|---|
1 | X1 | 32.768kHz crystal connection |
2 | X2 | 32.768kHz crystal connection |
3 | VBAT | +3V battery input for backup power |
4 | GND | Ground |
5 | SDA | Serial Data (I2C) |
6 | SCL | Serial Clock (I2C) |
7 | SQW/OUT | Square Wave/Output Driver |
8 | VCC | +5V main power supply |
X1 and X2 (Pins 1 and 2)
The X1 and X2 pins are used to connect a 32.768kHz crystal to the DS1307. This crystal provides the reference frequency for the IC’s oscillator circuit, enabling accurate timekeeping. When selecting a crystal, ensure that it has a load capacitance of 12.5pF, which is the standard value for the DS1307.
VBAT (Pin 3)
The VBAT pin is used to connect a backup battery to the DS1307. When the main power supply (VCC) is disconnected, the battery will maintain the IC’s timekeeping function and preserve the contents of the non-volatile RAM. The backup battery should provide a voltage between 2V and 3.5V. A common choice is a 3V lithium coin cell battery, such as the CR2032.
GND (Pin 4)
The GND pin is the ground connection for the DS1307. It should be connected to the common ground of your circuit.
SDA and SCL (Pins 5 and 6)
The SDA (Serial Data) and SCL (Serial Clock) pins are used for the I2C communication between the DS1307 and a microcontroller or other device. The I2C bus is a two-wire interface that allows multiple devices to communicate using just two lines: SDA for data transfer and SCL for synchronization.
To use the DS1307 with an I2C bus, you need to connect the SDA and SCL pins to the corresponding pins on your microcontroller. Most microcontrollers have dedicated I2C peripherals that can be configured to communicate with the DS1307. The I2C address of the DS1307 is fixed at 0x68 (or 1101000 in binary).
SQW/OUT (Pin 7)
The SQW/OUT pin is a programmable square-wave output that can be used for various purposes, such as generating interrupts or driving other devices. The square-wave frequency can be set to one of four values: 1Hz, 4kHz, 8kHz, or 32kHz. To enable the square-wave output, you need to set the SQWE bit in the DS1307’s control register.
If the square-wave output is not needed, the SQW/OUT pin can be left unconnected.
VCC (Pin 8)
The VCC pin is the main power supply input for the DS1307. It should be connected to a stable +5V power source. When the main power is disconnected, the DS1307 will automatically switch to battery backup mode, drawing power from the VBAT pin.
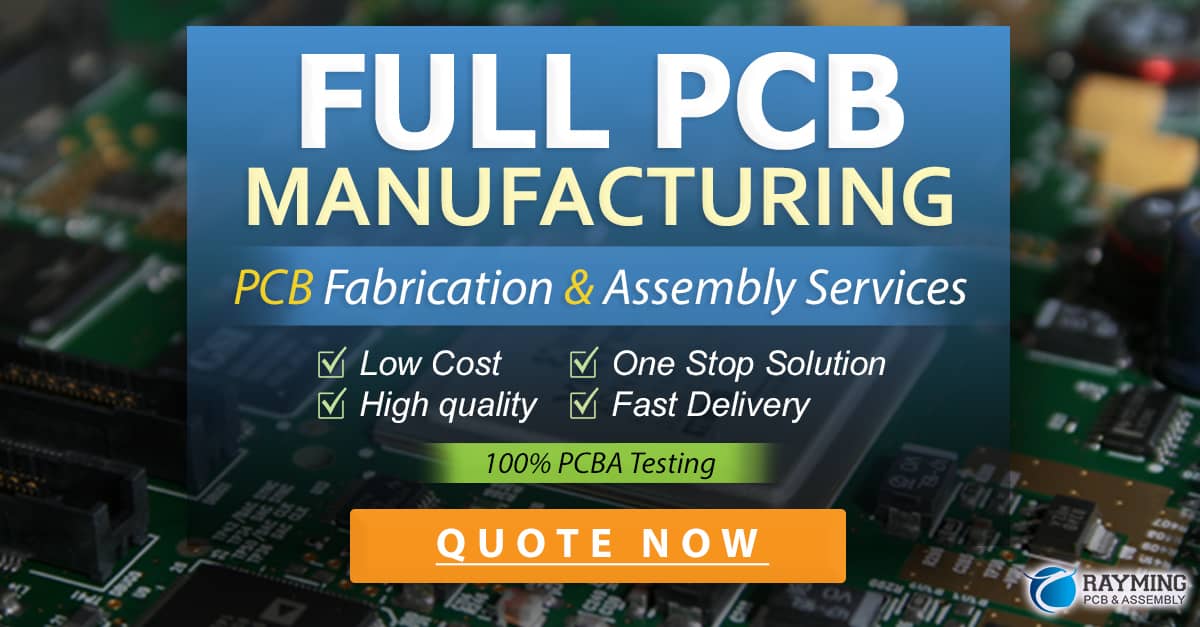
Interfacing the DS1307 with a Microcontroller
To integrate the DS1307 into your project, you’ll need to connect it to a microcontroller that supports I2C communication. Most popular microcontrollers, such as the Arduino and the Raspberry Pi, have libraries that make it easy to interface with the DS1307.
Here’s a simple example of how to connect the DS1307 to an Arduino Uno:
DS1307 Pin | Arduino Uno Pin |
---|---|
VCC | 5V |
GND | GND |
SDA | A4 (SDA) |
SCL | A5 (SCL) |
After connecting the DS1307 to your Arduino, you can use the RTClib library to set and read the time and date. Here’s a basic sketch that demonstrates how to set and read the time using the DS1307:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup () {
Serial.begin(9600);
rtc.begin();
// Set the time (uncomment the following lines if needed)
// rtc.adjust(DateTime(2023, 4, 18, 10, 30, 0));
}
void loop () {
DateTime now = rtc.now();
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(' ');
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.print(now.second(), DEC);
Serial.println();
delay(1000);
}
This sketch sets the initial time (if needed) in the setup() function and then continuously reads the current time and prints it to the serial monitor every second in the loop() function.
DS1307 Registers
The DS1307 has several internal registers that store the time, date, and configuration settings. These registers can be accessed and modified via the I2C interface.
The most important registers are:
- Seconds Register (00h): Stores the current seconds value in BCD format.
- Minutes Register (01h): Stores the current minutes value in BCD format.
- Hours Register (02h): Stores the current hours value in BCD format.
- Day Register (03h): Stores the current day of the week (1-7) in BCD format.
- Date Register (04h): Stores the current date of the month in BCD format.
- Month Register (05h): Stores the current month in BCD format.
- Year Register (06h): Stores the current year in BCD format.
- Control Register (07h): Used to control the square-wave output and other settings.
To set or read the time and date, you need to write to or read from these registers using the I2C interface. The DS1307 datasheet provides detailed information on the register formats and how to access them.
Troubleshooting Common Issues
If you encounter problems while using the DS1307, here are a few common issues and their solutions:
-
Incorrect time or date: If the DS1307 is displaying the wrong time or date, make sure that you have set the initial time correctly. Double-check the connections between the DS1307 and your microcontroller, and ensure that the I2C address is correct.
-
No communication with the DS1307: If your microcontroller cannot establish communication with the DS1307, check the following:
- Make sure that the SDA and SCL pins are connected correctly and are not swapped.
- Ensure that the DS1307 is powered correctly (VCC and GND connections).
- Check if the I2C bus is properly initialized in your microcontroller code.
-
Verify that the I2C address is correct (0x68 for the DS1307).
-
Battery backup not working: If the DS1307 does not maintain the time when the main power is disconnected, check the following:
- Ensure that the backup battery is connected correctly to the VBAT pin and GND.
- Verify that the battery voltage is within the acceptable range (2V to 3.5V).
- Check if the battery is depleted and needs to be replaced.
If you have checked all the above and the issue persists, it is possible that the DS1307 module is faulty. In this case, you may need to replace the module with a new one.
FAQs
-
Q: Can I use the DS1307 with a 3.3V microcontroller?
A: Yes, the DS1307 is compatible with both 3.3V and 5V microcontrollers. However, if you are using a 3.3V microcontroller, you need to ensure that the VCC pin of the DS1307 is connected to a 3.3V power source, and you may need to use level shifters for the SDA and SCL lines if your microcontroller’s I2C pins are not 5V tolerant. -
Q: How accurate is the DS1307?
A: The accuracy of the DS1307 depends on the quality of the crystal used and the operating temperature. With a standard 32.768kHz crystal, the DS1307 has a typical accuracy of ±2ppm at 25°C, which translates to about ±1 minute per year. However, the actual accuracy may vary depending on factors such as crystal quality, temperature fluctuations, and aging. -
Q: Can I use the DS1307 without a backup battery?
A: Yes, you can use the DS1307 without a backup battery. In this case, the timekeeping function will only work when the main power (VCC) is connected. When the main power is disconnected, the DS1307 will lose its time and date information, and you will need to set it again when power is restored. -
Q: How long does the backup battery last?
A: The backup battery life depends on factors such as the battery capacity, the operating temperature, and the DS1307’s power consumption. With a typical CR2032 lithium coin cell battery (225mAh capacity) and a DS1307 operating in battery backup mode, the battery can last for several years. However, it is recommended to replace the battery periodically to ensure uninterrupted timekeeping. -
Q: Can I connect multiple DS1307 modules to the same I2C bus?
A: No, you cannot connect multiple DS1307 modules to the same I2C bus because they all have the same fixed I2C address (0x68). If you need to use multiple RTC modules in your project, you can consider using other RTC ICs with different I2C addresses or using a separate I2C bus for each module.
Conclusion
The DS1307 is a versatile and reliable real-time clock IC that is widely used in various electronic projects. Its accurate timekeeping, low power consumption, and simple I2C interface make it an excellent choice for applications that require precise time and date information.
In this guide, we have covered the DS1307 pinout, its key features, and how to interface it with a microcontroller. We have also discussed common troubleshooting issues and provided answers to frequently asked questions.
By understanding the DS1307’s pinout and its functionality, you can easily integrate this powerful RTC module into your projects and build applications that rely on accurate timekeeping.
0 Comments