Introduction to the ESP-12E Wi-Fi Module
The ESP-12E Wi-Fi module is a popular, low-cost, and miniature solution for adding wireless connectivity to microcontroller projects. Developed by Espressif Systems, the ESP-12E module is based on the ESP8266 system-on-a-chip (SoC), which integrates a 32-bit Tensilica Xtensa LX106 microprocessor, Wi-Fi transceiver, and various peripherals. This module has gained significant attention in the IoT (Internet of Things) community due to its small form factor, low power consumption, and ease of use.
Key Features of the ESP-12E Wi-Fi Module
- Wi-Fi Connectivity:
- IEEE 802.11 b/g/n compliant
- Wi-Fi Direct (P2P) support
- Soft Access Point (AP) mode
- Station (STA) mode
-
WPA/WPA2 authentication and WEP/TKIP/AES encryption
-
Microcontroller:
- 32-bit Tensilica Xtensa LX106 CPU running at 80 MHz
- 64 KB of instruction RAM, 96 KB of data RAM
-
External QSPI flash support (up to 16 MB)
-
Peripherals:
- 16 GPIO pins
- SPI, I2C, UART, and I2S interfaces
- 10-bit ADC
-
PWM and IR remote control support
-
Low Power Consumption:
- Deep sleep mode consuming less than 10 μA
- Standby mode consuming less than 1.0 mA
-
Active mode consuming average 80 mA
-
Compact Form Factor:
- Dimensions: 16 mm x 24 mm x 3 mm
- SMD package with castellated edges for easy PCB mounting
Comparing the ESP-12E with Other Wi-Fi Modules
Module | Microcontroller | Wi-Fi Standard | Tx Power | Power Consumption (Active) | Size (mm) | Price (USD) |
---|---|---|---|---|---|---|
ESP-12E | ESP8266 | 802.11 b/g/n | 802.11 b/g/n | 80 mA | 16 x 24 x 3 | ~$2.00 |
ESP32 | ESP32 | 802.11 b/g/n | 802.11 b/g/n | 190 mA | 18 x 25.5 x 3.1 | ~$4.00 |
CC3200 | ARM Cortex-M4 | 802.11 b/g/n | 802.11 b/g/n | 230 mA | 18.92 x 30.48 x 2.5 | ~$9.00 |
RTL8710 | ARM Cortex-M3 | 802.11 b/g/n | 802.11 b/g/n | 86 mA | 17.6 x 26.7 x 2.3 | ~$3.00 |
The ESP-12E offers a balance between cost, performance, and size, making it an attractive choice for many IoT projects.
Getting Started with the ESP-12E Wi-Fi Module
Hardware Setup
To begin using the ESP-12E module, you’ll need the following components:
- ESP-12E Wi-Fi module
- USB-to-Serial adapter (e.g., FTDI FT232RL)
- 3.3V power supply
- Breadboard and jumper wires
- 10 kΩ resistor (for pulling up the CH_PD pin)
Follow these steps to set up the hardware:
- Connect the VCC pin of the ESP-12E to the 3.3V power supply.
- Connect the GND pin of the ESP-12E to the ground of the power supply and USB-to-Serial adapter.
- Connect the CH_PD pin of the ESP-12E to the 3.3V power supply through the 10 kΩ resistor.
- Connect the TXD pin of the ESP-12E to the RXD pin of the USB-to-Serial adapter.
- Connect the RXD pin of the ESP-12E to the TXD pin of the USB-to-Serial adapter.
Software Setup
To program the ESP-12E module, you can use the Arduino IDE with the ESP8266 core installed. Follow these steps to set up the software:
- Download and install the Arduino IDE from the official website: https://www.arduino.cc/en/software
- Open the Arduino IDE and navigate to File → Preferences.
- In the “Additional Boards Manager URLs” field, enter: http://arduino.esp8266.com/stable/package_esp8266com_index.json
- Click “OK” to save the preferences.
- Navigate to Tools → Board → Boards Manager.
- Search for “esp8266” and install the “esp8266 by ESP8266 Community” package.
- Select the “Generic ESP8266 Module” from Tools → Board.
You are now ready to start programming the ESP-12E Wi-Fi module using the Arduino IDE.
Programming the ESP-12E Wi-Fi Module
Basic Wi-Fi Connection Example
Here’s a simple example that demonstrates how to connect the ESP-12E to a Wi-Fi network and print the module’s IP address:
#include <ESP8266WiFi.h>
const char* ssid = "YourWiFiSSID";
const char* password = "YourWiFiPassword";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
// Your code here
}
Creating a Simple Web Server
The ESP-12E can also be used to create a simple web server that responds to client requests. Here’s an example:
#include <ESP8266WiFi.h>
const char* ssid = "YourWiFiSSID";
const char* password = "YourWiFiPassword";
WiFiServer server(80);
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
server.begin();
}
void loop() {
WiFiClient client = server.available();
if (client) {
Serial.println("New client connected");
while (client.connected()) {
if (client.available()) {
String request = client.readStringUntil('\r');
Serial.println(request);
client.println("HTTP/1.1 200 OK");
client.println("Content-type:text/html");
client.println();
client.println("<html><body>");
client.println("<h1>Hello from ESP-12E!</h1>");
client.println("</body></html>");
break;
}
}
client.stop();
Serial.println("Client disconnected");
}
}
This example creates a web server that listens on port 80 and responds to client requests with a simple HTML page.
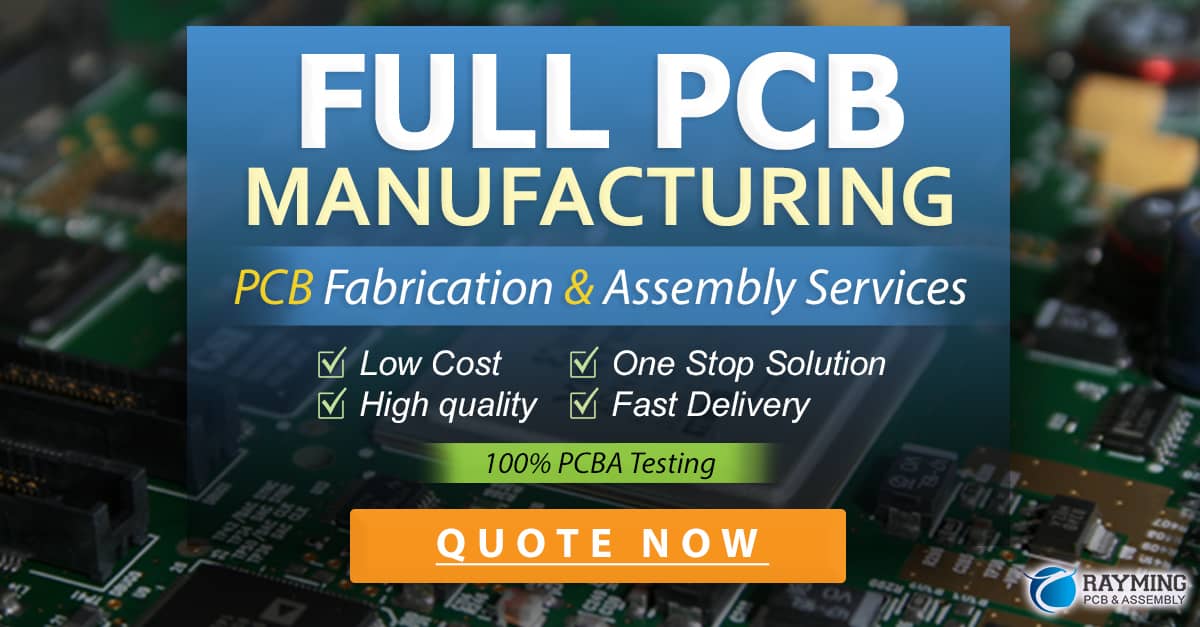
Advanced Topics and Applications
Over-the-Air (OTA) Updates
The ESP-12E supports over-the-air (OTA) updates, which allow you to update the firmware wirelessly without physically connecting the module to a computer. To enable OTA updates, you’ll need to include the ESP8266HTTPUpdateServer
library in your sketch and configure your web server to handle firmware uploads.
Here’s a basic example of how to set up OTA updates:
#include <ESP8266WiFi.h>
#include <ESP8266WebServer.h>
#include <ESP8266HTTPUpdateServer.h>
const char* ssid = "YourWiFiSSID";
const char* password = "YourWiFiPassword";
ESP8266WebServer server(80);
ESP8266HTTPUpdateServer httpUpdater;
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
httpUpdater.setup(&server);
server.begin();
}
void loop() {
server.handleClient();
}
To perform an OTA update, you’ll need to upload the new firmware using a web browser or a tool like cURL.
MQTT Communication
MQTT (Message Queuing Telemetry Transport) is a lightweight publish-subscribe messaging protocol commonly used in IoT applications. The ESP-12E can be used as an MQTT client to send and receive data from an MQTT broker.
To use MQTT with the ESP-12E, you’ll need to install the PubSubClient
library in the Arduino IDE. Here’s a basic example of how to connect to an MQTT broker and publish messages:
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
const char* ssid = "YourWiFiSSID";
const char* password = "YourWiFiPassword";
const char* mqttServer = "mqtt.example.com";
const int mqttPort = 1883;
WiFiClient espClient;
PubSubClient client(espClient);
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
client.setServer(mqttServer, mqttPort);
client.connect("ESP-12E");
}
void loop() {
if (!client.connected()) {
reconnect();
}
client.loop();
// Publish a message every 5 seconds
static unsigned long lastPublishTime = 0;
if (millis() - lastPublishTime >= 5000) {
lastPublishTime = millis();
client.publish("esp12e/status", "Hello from ESP-12E!");
}
}
void reconnect() {
while (!client.connected()) {
Serial.println("Connecting to MQTT broker...");
if (client.connect("ESP-12E")) {
Serial.println("Connected to MQTT broker");
} else {
Serial.print("Failed to connect to MQTT broker, rc=");
Serial.print(client.state());
Serial.println(" Retrying in 5 seconds");
delay(5000);
}
}
}
This example connects to an MQTT broker and publishes a message every 5 seconds. You can also subscribe to topics and receive messages from the broker by implementing the callback
function in the PubSubClient
library.
FAQ
-
What is the maximum range of the ESP-12E Wi-Fi module?
The range of the ESP-12E depends on various factors, such as the environment, antenna design, and transmission power. In ideal conditions (open space, line-of-sight), the ESP-12E can achieve a range of up to 100 meters. However, in indoor environments with obstacles, the range may be reduced to 20-30 meters. -
Can I use the ESP-12E as a standalone microcontroller without Wi-Fi?
Yes, the ESP-12E can be used as a standalone microcontroller without utilizing its Wi-Fi capabilities. The module has a 32-bit Tensilica Xtensa LX106 CPU that can be programmed to perform various tasks and interact with external sensors and actuators. -
How much power does the ESP-12E consume in different modes?
The power consumption of the ESP-12E varies depending on its operating mode: - Active mode (Wi-Fi enabled): ~80 mA
- Standby mode: <1.0 mA
-
Deep sleep mode: <10 μA
-
What is the flash memory size of the ESP-12E?
The ESP-12E module does not have built-in flash memory. However, it supports external QSPI flash memory up to 16 MB. The most common flash memory sizes used with the ESP-12E are 4 MB and 8 MB. -
Can I use the ESP-12E with batteries?
Yes, the ESP-12E can be powered by batteries. However, due to its relatively high power consumption in active mode, it is recommended to use power-saving techniques, such as deep sleep mode, to extend battery life. Additionally, ensure that the battery can supply the required current (peak current can reach up to 300 mA) and that the voltage is regulated to 3.3V.
Conclusion
The ESP-12E Wi-Fi module is a versatile and cost-effective solution for adding wireless connectivity to microcontroller projects. With its compact form factor, low power consumption, and extensive feature set, the ESP-12E is well-suited for a wide range of IoT applications, from home automation to industrial sensor networks.
By leveraging the Arduino IDE and the ESP8266 core, developers can quickly prototype and deploy ESP-12E-based projects using familiar programming concepts and libraries. The module’s support for over-the-air updates and MQTT communication further simplifies the development and maintenance of IoT devices.
As the IoT continues to grow and evolve, the ESP-12E Wi-Fi module remains a popular choice among hobbyists, researchers, and industry professionals alike. Its combination of affordability, performance, and ease of use makes it an ideal starting point for anyone looking to explore the world of connected devices and wireless communication.
0 Comments