Introduction to FPGA Programming
Field Programmable Gate Arrays (FPGAs) are powerful, flexible devices that allow you to create custom digital circuits. Unlike traditional integrated circuits, FPGAs can be reprogrammed after manufacturing, making them ideal for prototyping, experimenting, and implementing custom designs. In this article, we will explore the basics of FPGA programming and guide you through the process of programming an FPGA by yourself.
What is an FPGA?
An FPGA is an integrated circuit that consists of a matrix of configurable logic blocks (CLBs) connected via programmable interconnects. These CLBs can be configured to perform various logical functions, such as AND, OR, and NOT gates, as well as more complex functions like flip-flops, multiplexers, and memory elements. The programmable interconnects allow you to define the connections between the CLBs, creating a custom digital circuit.
Advantages of FPGAs
FPGAs offer several advantages over traditional integrated circuits:
- Flexibility: FPGAs can be reprogrammed to implement different designs, making them suitable for a wide range of applications.
- Rapid prototyping: With FPGAs, you can quickly test and iterate on your designs without the need for costly and time-consuming fabrication processes.
- Parallel processing: FPGAs can perform multiple tasks simultaneously, enabling high-performance parallel processing.
- Energy efficiency: FPGAs consume less power compared to general-purpose processors for certain applications.
Getting Started with FPGA Programming
To begin programming an FPGA, you will need the following:
- An FPGA development board
- A computer with an FPGA development software installed
- A programming language (such as Verilog or VHDL) or a high-level synthesis tool
Choosing an FPGA Development Board
There are numerous FPGA development boards available in the market, catering to different skill levels and application requirements. Some popular options include:
Board | Manufacturer | FPGA Chip | Key Features |
---|---|---|---|
Basys 3 | Digilent | Xilinx Artix-7 | Beginner-friendly, on-board switches, LEDs, and VGA output |
DE10-Lite | Terasic | Intel MAX 10 | Low-cost, on-board accelerometer, GPIO, and Arduino headers |
Arty A7 | Digilent | Xilinx Artix-7 | Ethernet, USB, and HDMI interfaces, on-board DDR3 memory |
Cyclone V GX Starter Kit | Intel | Intel Cyclone V | PCIe, Ethernet, and DDR3 memory interfaces, on-board USB-Blaster II |
When selecting an FPGA development board, consider factors such as the FPGA chip, available peripherals, and your budget.
Installing FPGA Development Software
To program an FPGA, you will need an Integrated Development Environment (IDE) that supports your chosen FPGA chip and programming language. The two major FPGA manufacturers, Xilinx and Intel, provide their own development software:
- Xilinx: Vivado Design Suite (for Artix, Kintex, and Virtex FPGAs) and ISE Design Suite (for older Spartan FPGAs)
- Intel: Quartus Prime (for Cyclone, Arria, and Stratix FPGAs)
These IDEs include tools for design entry, synthesis, simulation, and programming the FPGA.
Choosing a Programming Language
FPGAs can be programmed using hardware description languages (HDLs) such as Verilog and VHDL. These languages allow you to describe the behavior and structure of your digital circuit. Alternatively, you can use high-level synthesis (HLS) tools that convert high-level languages like C, C++, or SystemC into HDL code.
If you are new to FPGA programming, Verilog is often recommended as a starting point due to its simpler syntax and easier learning curve compared to VHDL.
FPGA Programming Flow
The typical FPGA programming flow consists of the following steps:
- Design entry: Create your digital circuit using an HDL or HLS tool.
- Synthesis: Convert the HDL code into a netlist, which represents the circuit in terms of the FPGA’s available resources.
- Implementation: Map the netlist onto the FPGA’s physical resources, optimize the design, and generate a bitstream.
- Programming: Load the bitstream onto the FPGA to configure its CLBs and interconnects.
Design Entry
During the design entry phase, you will write HDL code to describe your digital circuit. This involves defining the inputs, outputs, and the behavior of your circuit using constructs like modules, always blocks, and assignments.
Here’s a simple example of a Verilog module that implements an AND gate:
module and_gate (
input a,
input b,
output y
);
assign y = a & b;
endmodule
Synthesis
The synthesis process takes your HDL code and translates it into a netlist, which is a representation of your circuit in terms of the FPGA’s available resources. The synthesis tool optimizes the design to minimize the use of resources and improve performance.
To perform synthesis, you will need to create a project in your FPGA development software, add your HDL files, and configure the synthesis settings. The specific steps may vary depending on your chosen IDE.
Implementation
During the implementation phase, the netlist is mapped onto the FPGA’s physical resources, such as CLBs, I/O blocks, and interconnects. This process involves several sub-steps:
- Translate: Convert the netlist into a format suitable for the target FPGA architecture.
- Map: Assign the netlist elements to the FPGA’s physical resources.
- Place and Route: Determine the optimal placement of the mapped resources and route the connections between them.
- Bitstream Generation: Generate a binary file (bitstream) that contains the configuration data for the FPGA.
The implementation process is typically automated by the FPGA development software, but you may need to adjust settings to optimize performance or meet specific constraints.
Programming
To program the FPGA, you will need to connect your development board to your computer and use the FPGA development software to load the bitstream onto the device. This process is called configuration.
Most FPGA development boards have a JTAG (Joint Test Action Group) interface for programming. The specific steps to program the FPGA will depend on your development board and IDE, but generally involve the following:
- Connect the FPGA board to your computer using a USB or JTAG cable.
- Open your project in the FPGA development software.
- Configure the programming settings, specifying the target device and bitstream file.
- Initiate the programming process, which will transfer the bitstream to the FPGA.
Once the FPGA is programmed, it will retain its configuration until it is reprogrammed or powered off.
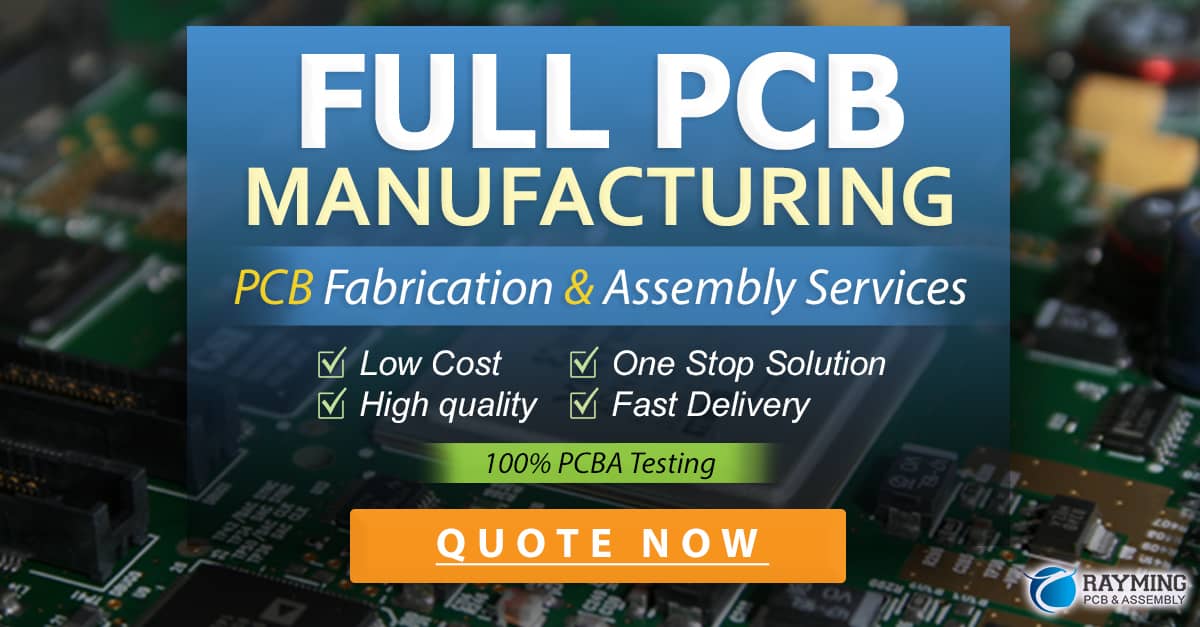
Example: Blinking LED
Let’s walk through a simple example of programming an FPGA to blink an LED. We will use a Basys 3 board with a Xilinx Artix-7 FPGA and Verilog as our programming language.
Step 1: Design Entry
Create a new Verilog file named blink.v
and enter the following code:
module blink (
input clk,
output led
);
reg [24:0] counter;
always @(posedge clk) begin
counter <= counter + 1;
end
assign led = counter[24];
endmodule
This module uses a 25-bit counter to divide the clock frequency and toggle the LED state every 2^24 clock cycles.
Step 2: Synthesis
- Open Xilinx Vivado and create a new project.
- Add the
blink.v
file to the project. - Set the target device to the Artix-7 FPGA on the Basys 3 board.
- Run the synthesis process.
Step 3: Implementation
- In Vivado, run the implementation process, which includes translation, mapping, and place and route.
- Generate the bitstream file.
Step 4: Programming
- Connect the Basys 3 board to your computer using a USB cable.
- In Vivado, open the Hardware Manager and select the target device.
- Program the FPGA with the generated bitstream.
Once programmed, you should see the LED on the Basys 3 board blinking at a steady rate.
Conclusion
FPGA programming allows you to create custom digital circuits and unleash your creativity in hardware design. By following the steps outlined in this article, you can start programming FPGAs by yourself and explore the vast possibilities they offer.
Remember to choose an appropriate FPGA development board, install the necessary software, and familiarize yourself with the programming language and flow. With practice and persistence, you can master FPGA programming and bring your unique ideas to life.
FAQ
-
Q: What is the difference between Verilog and VHDL?
A: Verilog and VHDL are both hardware description languages used for FPGA programming. Verilog has a simpler syntax and is more popular in the United States, while VHDL is more verbose and is more common in Europe. Both languages offer similar capabilities, and the choice often depends on personal preference or project requirements. -
Q: Can I use high-level languages like C or Python for FPGA programming?
A: Yes, you can use high-level synthesis (HLS) tools to convert high-level languages like C, C++, or SystemC into HDL code. Some FPGA vendors also provide tools that allow you to use Python for certain aspects of FPGA programming. However, for optimal performance and control, it is often necessary to work with HDLs directly. -
Q: How do I choose the right FPGA for my project?
A: When selecting an FPGA, consider factors such as the required logic capacity, performance, power consumption, and available I/O interfaces. Evaluate your project requirements and consult the FPGA vendor’s device selection guides to find a suitable device. It’s also important to consider the cost and the availability of development tools and support for the chosen FPGA family. -
Q: What are some common applications of FPGAs?
A: FPGAs are used in a wide range of applications, including: - Digital signal processing (DSP)
- Automotive and aerospace systems
- Medical devices
- Industrial control and automation
- Computer vision and image processing
- Cryptography and security
-
High-performance computing and data centers
-
Q: Are there any open-source tools for FPGA programming?
A: Yes, there are several open-source tools and frameworks available for FPGA programming: - Icarus Verilog: A Verilog simulation and synthesis tool
- Yosys: An open-source hardware synthesis tool for Verilog
- Project IceStorm: An open-source toolchain for Lattice iCE40 FPGAs
- LiteX: A Python-based framework for building FPGA-based systems
These tools provide alternatives to the proprietary software offered by FPGA vendors, although they may have limitations in terms of device support and features.
0 Comments