Introduction to 4×4 Keypads
A 4×4 matrix keypad is a simple input device commonly used in electronic projects to interface with microcontrollers or other circuits. It consists of 16 buttons arranged in a 4 by 4 grid, allowing users to input numbers, letters, or custom commands. 4×4 keypads are widely used in applications such as:
- Access control systems
- Calculator interfaces
- Industrial control panels
- Interactive projects
In this comprehensive guide, we will dive deep into the world of 4×4 keypads, exploring their working principle, wiring, programming, and practical applications. Whether you are a beginner looking to incorporate a keypad into your project or an experienced developer seeking to expand your knowledge, this article will provide you with valuable insights and hands-on examples.
How a 4×4 Keypad Works
Matrix Circuit
A 4×4 keypad operates on a matrix circuit principle. The 16 buttons are arranged in a grid of 4 rows and 4 columns. Each button is connected to a unique combination of a row and a column pin. When a button is pressed, it closes the circuit between the corresponding row and column, allowing the microcontroller to detect which button was activated.
The matrix arrangement reduces the number of I/O pins required to interface the keypad with a microcontroller. Instead of using 16 separate pins for each button, a 4×4 keypad requires only 8 pins (4 rows and 4 columns) to scan and detect button presses.
Keypad Scanning
To determine which button is pressed, the microcontroller performs a scanning process. It follows these steps:
- Set all column pins as OUTPUT and all row pins as INPUT_PULLUP.
- Set one column pin to LOW and others to HIGH.
- Check the status of each row pin sequentially.
- If a row pin reads LOW, the button at the intersection of the active column and row is pressed.
- If a row pin reads HIGH, no button is pressed in that row for the active column.
- Repeat steps 2-3 for each column pin.
By cycling through each column and checking the status of the row pins, the microcontroller can identify the specific button that was pressed.
Button Debouncing
When a button is pressed or released, mechanical contacts may bounce, causing multiple transitions between open and closed states. This bouncing can lead to false readings and unintended behavior in the microcontroller.
To mitigate this issue, button debouncing techniques are employed. Debouncing ensures that only a single button press is registered, even if the physical button bounces. Common debouncing methods include:
- Software debouncing: Implementing a delay or a waiting period in the code to ignore rapid button state changes.
- Hardware debouncing: Adding a capacitor or a resistor-capacitor (RC) circuit to filter out the bouncing signals.
Proper debouncing is crucial for reliable keypad functionality and preventing erroneous inputs.
Wiring a 4×4 Keypad
Wiring a 4×4 keypad to a microcontroller is a straightforward process. Most 4×4 keypads have 8 pins: 4 row pins and 4 column pins. The pinout of a typical 4×4 keypad is as follows:
Pin | Connection |
---|---|
1 | Row 1 |
2 | Row 2 |
3 | Row 3 |
4 | Row 4 |
5 | Column 1 |
6 | Column 2 |
7 | Column 3 |
8 | Column 4 |
To connect the keypad to a microcontroller, follow these steps:
- Identify the row and column pins on the keypad.
- Connect each row pin of the keypad to a digital I/O pin of the microcontroller. Enable the internal pull-up resistors for these pins.
- Connect each column pin of the keypad to a digital I/O pin of the microcontroller.
- Ensure a common ground connection between the keypad and the microcontroller.
Here’s an example wiring diagram for connecting a 4×4 keypad to an Arduino:
Keypad Arduino
-----------------
Row 1 -> Pin 9
Row 2 -> Pin 8
Row 3 -> Pin 7
Row 4 -> Pin 6
Col 1 -> Pin 5
Col 2 -> Pin 4
Col 3 -> Pin 3
Col 4 -> Pin 2
Once the wiring is complete, you can proceed to program the microcontroller to scan the keypad and detect button presses.
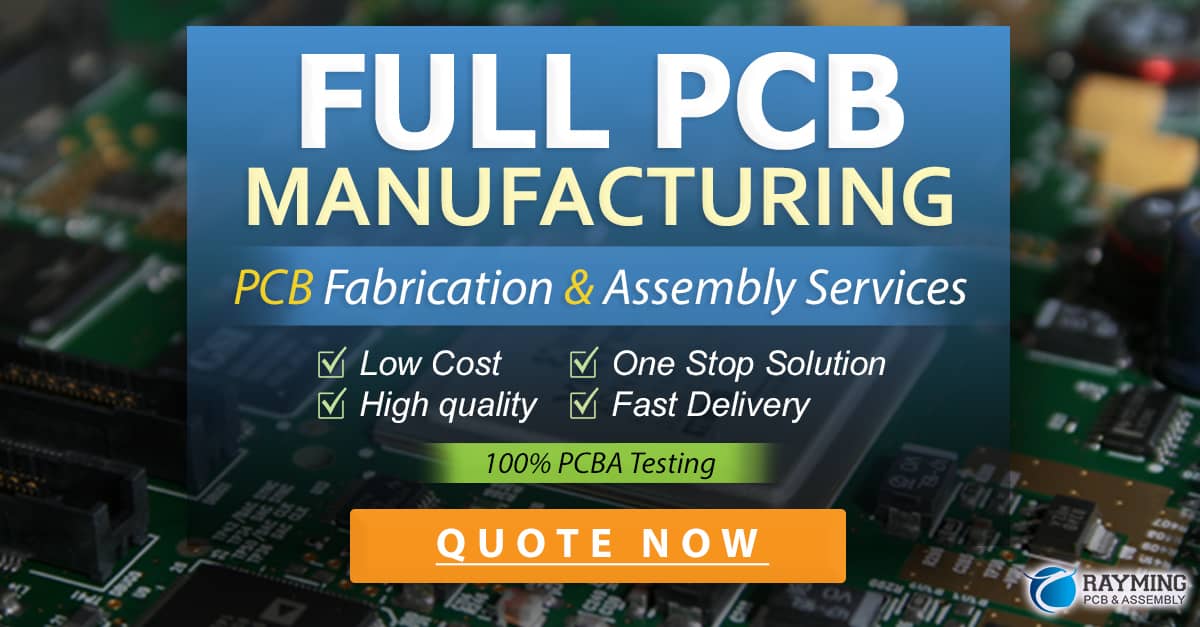
Programming a 4×4 Keypad
Programming a 4×4 keypad involves scanning the matrix and interpreting the button presses. Here’s a step-by-step guide to programming a 4×4 keypad with an Arduino:
-
Install the Keypad Library: The Keypad library simplifies the process of interfacing with a 4×4 keypad. You can install it through the Arduino IDE’s Library Manager.
-
Include the Keypad Library: In your Arduino sketch, include the Keypad library at the top:
cpp
#include <Keypad.h> -
Define the Keypad Layout: Create a 2D array representing the layout of the keypad buttons. For example:
cpp
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
}; -
Define the Pin Connections: Specify the Arduino pins connected to the keypad’s row and column pins:
cpp
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2}; -
Create a Keypad Object: Instantiate a Keypad object with the defined key layout and pin connections:
cpp
Keypad keypad = Keypad(makeKeymap(keys), rowPins, colPins, ROWS, COLS); -
Check for Key Presses: In the
loop()
function, use thekeypad.getKey()
method to check for key presses:
cpp
void loop() {
char key = keypad.getKey();
if (key) {
// Process the pressed key
// ...
}
} -
Process the Pressed Key: When a key is pressed, you can perform actions based on the pressed key. For example, you can display the pressed key on the serial monitor:
cpp
if (key) {
Serial.print("Pressed: ");
Serial.println(key);
}
This is a basic example of programming a 4×4 keypad with Arduino. You can customize the key layout, add more complex functions, or integrate the keypad input with other parts of your project based on your specific requirements.
Practical Applications of 4×4 Keypads
4×4 keypads find applications in various projects and systems. Let’s explore a few practical examples:
Access Control System
A 4×4 keypad can be used as an input device for an access control system. Users can enter a passcode or PIN to unlock a door or grant access to a restricted area. Here’s a basic implementation:
- Connect the 4×4 keypad to a microcontroller (e.g., Arduino).
- Define a secret passcode or PIN in the code.
- Prompt the user to enter the passcode using the keypad.
- Compare the entered passcode with the predefined secret passcode.
- If the passcode is correct, activate a relay or send a signal to unlock the door.
- If the passcode is incorrect, display an error message or trigger an alarm.
By using a 4×4 keypad, you can create a simple yet effective access control system for homes, offices, or secure facilities.
Calculator Interface
A 4×4 keypad is commonly used as an input device for calculator projects. You can create a basic calculator by interfacing a keypad with a microcontroller and a display module (e.g., LCD or OLED). Here’s a step-by-step guide:
- Connect the 4×4 keypad and display module to the microcontroller.
- Initialize the keypad and display in the code.
- Continuously scan for key presses using the keypad library.
- When a key is pressed, determine its function (digit, operator, or special command).
- Update the display based on the pressed key and the current state of the calculator.
- Perform the corresponding calculation when the equals (=) key is pressed.
- Display the result on the screen.
By combining a 4×4 keypad, microcontroller, and display, you can create a functional calculator for basic arithmetic operations.
Interactive Projects
4×4 keypads can add interactivity to various projects, allowing users to input commands, select options, or navigate menus. Here are a few examples:
-
Menu Navigation: Use the keypad to navigate through a menu system displayed on an LCD or OLED screen. Assign specific keys for moving up, down, selecting options, or going back.
-
Game Controller: Create simple games controlled by a 4×4 keypad. Use the keys to move a character, select actions, or input game commands.
-
Remote Control: Use a keypad to send commands wirelessly to control devices or robots. Assign different keys to specific actions or movements.
-
Data Entry: Implement a data entry system where users can input numeric or alphanumeric data using the keypad. Display the entered data on a screen or store it for further processing.
The possibilities are endless when it comes to interactive projects with 4×4 keypads. By leveraging the keypad’s input capabilities, you can create engaging and user-friendly interfaces for your projects.
Frequently Asked Questions (FAQ)
-
Q: Can I use a 4×4 keypad with any microcontroller?
A: Yes, you can use a 4×4 keypad with most microcontrollers that have sufficient digital I/O pins. Popular choices include Arduino, Raspberry Pi, and various other development boards. -
Q: How do I handle multiple simultaneous button presses on a 4×4 keypad?
A: Handling multiple simultaneous button presses requires a more advanced scanning technique called “ghosting” prevention. It involves using diodes or a specialized matrix keypad controller to eliminate the ghosting effect and accurately detect multiple key presses. -
Q: Can I customize the keypad layout or use different characters?
A: Yes, you can customize the keypad layout by modifying the 2D array that represents the key characters. You can assign any character, symbol, or custom value to each button position in the array. -
Q: How can I improve the responsiveness of the keypad?
A: To improve the responsiveness of the keypad, you can adjust the debounce delay in the code. Experiment with different delay values to find the optimal balance between responsiveness and eliminating false readings. Additionally, ensure proper wiring and consider using hardware debouncing techniques if necessary. -
Q: Are there any ready-made keypad libraries for Arduino or other platforms?
A: Yes, there are several keypad libraries available for Arduino and other platforms. The most popular one is the Keypad library for Arduino, which simplifies the process of interfacing with matrix keypads. Other platforms like Raspberry Pi also have similar libraries available.
Conclusion
In this comprehensive guide, we explored the world of 4×4 keypads, covering their working principle, wiring, programming, and practical applications. We learned how the matrix circuit enables efficient scanning of button presses and discussed techniques like button debouncing to ensure reliable functionality.
We also delved into the process of wiring a 4×4 keypad to a microcontroller and provided step-by-step instructions for programming it using the Arduino platform. Additionally, we explored various practical applications, including access control systems, calculator interfaces, and interactive projects.
By understanding the concepts and techniques presented in this guide, you can confidently incorporate 4×4 keypads into your projects and create intuitive user interfaces. Whether you are building a simple keypad-controlled system or a complex interactive project, the versatility and ease of use of 4×4 keypads make them a valuable addition to your toolkit.
Remember to experiment, customize, and adapt the code and wiring to suit your specific project requirements. With a solid foundation in 4×4 keypads, you can unlock a world of possibilities and bring your ideas to life.
Happy keypad hacking!
0 Comments