What is a Stepper Motor?
A stepper motor is a type of DC motor that moves in precise increments, or steps, allowing for accurate position control. Unlike continuous rotation motors, stepper motors can be controlled to rotate a specific number of degrees, making them ideal for applications requiring precise positioning, such as 3D printers, CNC machines, and robotics.
Advantages of Stepper Motors
- Precise position control
- High torque at low speeds
- Excellent repeatability
- No feedback sensor required for positioning
- Low cost compared to servo motors
The 28BYJ-48 Stepper Motor
The 28BYJ-48 is a Unipolar Stepper motor with four phases, each requiring a separate control wire. It typically comes with a ULN2003 driver board, which simplifies the wiring and control of the motor.
28BYJ-48 Specifications
Specification | Value |
---|---|
Rated Voltage | 5V DC |
Number of Phases | 4 |
Stride Angle | 5.625° / 64 |
Steps per Revolution | 64 |
Frequency | 100 Hz |
DC Resistance | 50 Ω ±7% (25°C) |
Idle In-traction Frequency | > 600 Hz |
Idle Out-traction Frequency | > 1000 Hz |
In-traction Torque | > 34.3 mN.m (120 Hz) |
Self-positioning Torque | > 34.3 mN.m |
Friction Torque | 600-1200 gf.cm |
Pull-in Torque | 300 gf.cm |
Insulated Resistance | > 10 MΩ (500V) |
Insulated Electricity Power | 600 VAC / 1 mA / 1 s |
Insulation Grade | A |
Rise in Temperature | < 40 K (120 Hz) |
Noise | < 35 dB (120 Hz, no load, 10 cm) |
Wiring the 28BYJ-48 to Arduino
To control the 28BYJ-48 stepper motor with Arduino, you’ll need to connect the ULN2003 driver board to the Arduino’s digital pins. The driver board has six pins: four for the motor phases and two for power.
Connection Table
ULN2003 Board | Arduino |
---|---|
IN1 | Digital Pin 8 |
IN2 | Digital Pin 9 |
IN3 | Digital Pin 10 |
IN4 | Digital Pin 11 |
+ | 5V |
– | GND |
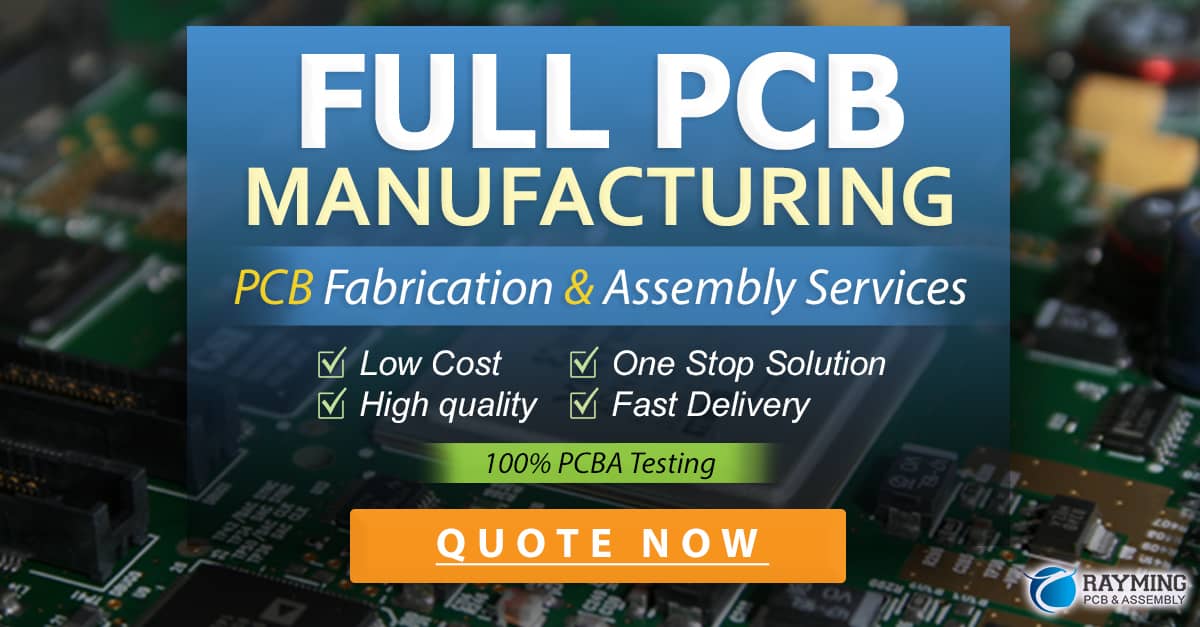
Programming the 28BYJ-48 with Arduino
To control the 28BYJ-48 stepper motor with Arduino, you can use the Arduino Stepper library or create your own functions using the digitalWrite() function.
Using the Arduino Stepper Library
- Include the Stepper library in your Arduino sketch:
#include <Stepper.h>
- Define the number of steps per revolution and the stepper object:
const int stepsPerRevolution = 2048; // Change this to match your motor
Stepper myStepper(stepsPerRevolution, 8, 10, 9, 11);
- Set the motor speed in the setup() function:
void setup() {
myStepper.setSpeed(10); // 10 rpm
}
- Control the motor in the loop() function:
void loop() {
myStepper.step(stepsPerRevolution); // Rotate one revolution clockwise
delay(500);
myStepper.step(-stepsPerRevolution); // Rotate one revolution counterclockwise
delay(500);
}
Creating Custom Functions
- Define the pin numbers connected to the ULN2003 board:
const int IN1 = 8;
const int IN2 = 9;
const int IN3 = 10;
const int IN4 = 11;
- Create an array of step sequences:
const int stepsPerRevolution = 2048;
int stepSequence[8][4] = {
{1, 0, 0, 0},
{1, 1, 0, 0},
{0, 1, 0, 0},
{0, 1, 1, 0},
{0, 0, 1, 0},
{0, 0, 1, 1},
{0, 0, 0, 1},
{1, 0, 0, 1}
};
- Initialize the pins as outputs in the setup() function:
void setup() {
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
}
- Create functions to rotate the motor clockwise and counterclockwise:
void clockwise(int steps) {
for (int i = 0; i < steps; i++) {
for (int j = 0; j < 8; j++) {
digitalWrite(IN1, stepSequence[j][0]);
digitalWrite(IN2, stepSequence[j][1]);
digitalWrite(IN3, stepSequence[j][2]);
digitalWrite(IN4, stepSequence[j][3]);
delay(2);
}
}
}
void counterclockwise(int steps) {
for (int i = 0; i < steps; i++) {
for (int j = 7; j >= 0; j--) {
digitalWrite(IN1, stepSequence[j][0]);
digitalWrite(IN2, stepSequence[j][1]);
digitalWrite(IN3, stepSequence[j][2]);
digitalWrite(IN4, stepSequence[j][3]);
delay(2);
}
}
}
- Call the functions in the loop() to control the motor:
void loop() {
clockwise(stepsPerRevolution); // Rotate one revolution clockwise
delay(500);
counterclockwise(stepsPerRevolution); // Rotate one revolution counterclockwise
delay(500);
}
Practical Examples
Controlling the 28BYJ-48 with a Potentiometer
- Connect a potentiometer to analog pin A0.
- Read the potentiometer value and map it to the number of steps:
int potValue = analogRead(A0);
int steps = map(potValue, 0, 1023, -stepsPerRevolution, stepsPerRevolution);
- Use the steps value to control the motor direction and speed:
if (steps > 0) {
clockwise(steps);
} else if (steps < 0) {
counterclockwise(abs(steps));
}
Creating a Simple Robot with the 28BYJ-48
- Connect two 28BYJ-48 motors to the Arduino, one for each wheel.
- Define the pin numbers and create stepper objects for both motors:
const int stepsPerRevolution = 2048;
Stepper leftStepper(stepsPerRevolution, 2, 4, 3, 5);
Stepper rightStepper(stepsPerRevolution, 8, 10, 9, 11);
- Create functions to move the robot forward, backward, left, and right:
void moveForward(int steps) {
leftStepper.step(steps);
rightStepper.step(steps);
}
void moveBackward(int steps) {
leftStepper.step(-steps);
rightStepper.step(-steps);
}
void turnLeft(int steps) {
leftStepper.step(-steps);
rightStepper.step(steps);
}
void turnRight(int steps) {
leftStepper.step(steps);
rightStepper.step(-steps);
}
- Use the functions to control the robot’s movement in the loop() function:
void loop() {
moveForward(stepsPerRevolution); // Move forward one rotation
delay(500);
turnLeft(stepsPerRevolution / 4); // Turn left 90 degrees
delay(500);
moveBackward(stepsPerRevolution); // Move backward one rotation
delay(500);
turnRight(stepsPerRevolution / 4); // Turn right 90 degrees
delay(500);
}
FAQ
-
Q: Can I use the 28BYJ-48 stepper motor without the ULN2003 driver board?
A: While it is possible to control the 28BYJ-48 without the ULN2003 driver board, it is not recommended. The driver board simplifies the wiring and provides the necessary current amplification to drive the motor efficiently. -
Q: How can I increase the torque of the 28BYJ-48 stepper motor?
A: To increase the torque of the 28BYJ-48, you can try the following: - Increase the current supplied to the motor by using an external power source.
- Use a gear reduction system to increase the torque at the cost of speed.
-
Use multiple 28BYJ-48 motors in parallel to combine their torque output.
-
Q: What is the maximum speed I can achieve with the 28BYJ-48 stepper motor?
A: The maximum speed of the 28BYJ-48 depends on factors such as the supply voltage, driver board, and load. In general, the motor can achieve speeds up to 15 RPM (revolutions per minute) when driven at its rated voltage of 5V. -
Q: Can I control multiple 28BYJ-48 stepper motors with a single Arduino board?
A: Yes, you can control multiple 28BYJ-48 stepper motors with a single Arduino board. However, keep in mind that each motor requires four digital pins for control. Make sure your Arduino has enough digital pins to accommodate all the motors you wish to control. -
Q: Are there any libraries available for controlling the 28BYJ-48 with Arduino?
A: Yes, there are several libraries available for controlling the 28BYJ-48 with Arduino, such as: - AccelStepper: A powerful library that supports acceleration and deceleration control.
- Arduino Stepper: The official Arduino library for controlling stepper motors.
- Stepper28BYJ48: A library specifically designed for the 28BYJ-48 stepper motor.
Conclusion
The 28BYJ-48 stepper motor is a versatile and affordable choice for Arduino-based projects requiring precise position control. By following the wiring and programming examples provided in this article, you can easily integrate the 28BYJ-48 into your projects and create a wide range of applications, from simple position control to complex robotics. Remember to experiment, learn, and have fun while exploring the possibilities of the 28BYJ-48 stepper motor with Arduino!
0 Comments